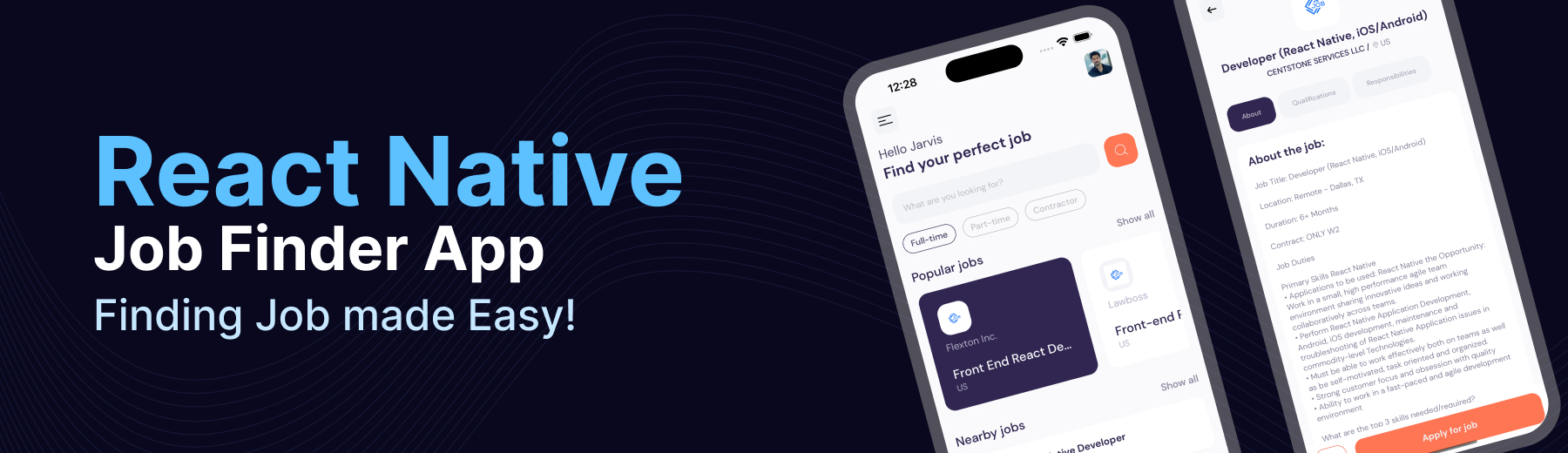
π Table of Contents
- π€ Introduction
- βοΈ Tech Stack
- π Features
- π€Έ Quick Start
- πΈοΈ Snippets
Discover the power of React Native and Expo with my job-search application. Crafted for a seamless user experience, the app boasts visually appealing UI/UX, third-party API integration, and dynamic features like search, pagination, and custom hooks. From a responsive design to detailed job insights, this project epitomizes React Native development, showcasing advanced skills in Node.js, Axios, and stylesheet management. Dive into a world of code architecture and reusability, offering a comprehensive hands-on experience in building a feature-rich app for job exploration and application.
- Node.js
- React Native
- Axios
- Expo
- Stylesheet
π Visually Appealing UI/UX Design: Develop an aesthetically pleasing user interface using React Native components.
π Third Party API Integration: Fetch data from an external API and seamlessly integrate it into the app.
π Search & Pagination Functionality: Implement search functionality and pagination for efficient data navigation.
π Custom API Data Fetching Hooks:Create custom hooks for streamlined and reusable API data fetching.
π Dynamic Home Page: Explore diverse jobs from popular and nearby locations across different categories.
π Browse with Ease on Explore Page: Page: Navigate through various jobs spanning different categories and types.
π Detailed Job Insights: View comprehensive job details, including application links, salary info, responsibilities, and qualifications.
π Tailored Job Exploration: Find jobs specific to a particular title
π Robust Loading and Error Management: Ensure effective handling of loading processes and error scenarios.
π Optimized for All Devices: A responsive design for a seamless user experience across various devices.
and many more, including code architecture and reusability
Follow these steps to set up the project locally on your machine.
Prerequisites
Make sure you have the following installed on your machine:
Cloning the Repository
git clone https://github.com/adrianhajdin/project_react_native_jobs.git
cd project_react_native_jobs
Installation
Install the project dependencies using npm:
npm install
Set Up Environment Variables
Create a new file named .env
in the root of your project and add the following content:
X-RapidAPI-Key=
Replace the placeholder values with your actual credentials. You can obtain these credentials by signing up on the RapidAPI website.
Running the Project
npm start
Open http://localhost:3000 in your browser to view the project.
Search.js
import React, { useEffect, useState } from 'react'
import { ActivityIndicator, FlatList, Image, TouchableOpacity, View } from 'react-native'
import { Stack, useRouter, useSearchParams } from 'expo-router'
import { Text, SafeAreaView } from 'react-native'
import axios from 'axios'
import { ScreenHeaderBtn, NearbyJobCard } from '../../components'
import { COLORS, icons, SIZES } from '../../constants'
import styles from '../../styles/search'
const JobSearch = () => {
const params = useSearchParams();
const router = useRouter()
const [searchResult, setSearchResult] = useState([]);
const [searchLoader, setSearchLoader] = useState(false);
const [searchError, setSearchError] = useState(null);
const [page, setPage] = useState(1);
const handleSearch = async () => {
setSearchLoader(true);
setSearchResult([])
try {
const options = {
method: "GET",
url: `https://jsearch.p.rapidapi.com/search`,
headers: {
"X-RapidAPI-Key": '',
"X-RapidAPI-Host": "jsearch.p.rapidapi.com",
},
params: {
query: params.id,
page: page.toString(),
},
};
const response = await axios.request(options);
setSearchResult(response.data.data);
} catch (error) {
setSearchError(error);
console.log(error);
} finally {
setSearchLoader(false);
}
};
const handlePagination = (direction) => {
if (direction === 'left' && page > 1) {
setPage(page - 1)
handleSearch()
} else if (direction === 'right') {
setPage(page + 1)
handleSearch()
}
}
useEffect(() => {
handleSearch()
}, [])
return (
<SafeAreaView style={{ flex: 1, backgroundColor: COLORS.lightWhite }}>
<Stack.Screen
options={{
headerStyle: { backgroundColor: COLORS.lightWhite },
headerShadowVisible: false,
headerLeft: () => (
<ScreenHeaderBtn
iconUrl={icons.left}
dimension='60%'
handlePress={() => router.back()}
/>
),
headerTitle: "",
}}
/>
<FlatList
data={searchResult}
renderItem={({ item }) => (
<NearbyJobCard
job={item}
handleNavigate={() => router.push(`/job-details/${item.job_id}`)}
/>
)}
keyExtractor={(item) => item.job_id}
contentContainerStyle={{ padding: SIZES.medium, rowGap: SIZES.medium }}
ListHeaderComponent={() => (
<>
<View style={styles.container}>
<Text style={styles.searchTitle}>{params.id}</Text>
<Text style={styles.noOfSearchedJobs}>Job Opportunities</Text>
</View>
<View style={styles.loaderContainer}>
{searchLoader ? (
<ActivityIndicator size='large' color={COLORS.primary} />
) : searchError && (
<Text>Oops something went wrong</Text>
)}
</View>
</>
)}
ListFooterComponent={() => (
<View style={styles.footerContainer}>
<TouchableOpacity
style={styles.paginationButton}
onPress={() => handlePagination('left')}
>
<Image
source={icons.chevronLeft}
style={styles.paginationImage}
resizeMode="contain"
/>
</TouchableOpacity>
<View style={styles.paginationTextBox}>
<Text style={styles.paginationText}>{page}</Text>
</View>
<TouchableOpacity
style={styles.paginationButton}
onPress={() => handlePagination('right')}
>
<Image
source={icons.chevronRight}
style={styles.paginationImage}
resizeMode="contain"
/>
</TouchableOpacity>
</View>
)}
/>
</SafeAreaView>
)
}
export default JobSearch