-
Notifications
You must be signed in to change notification settings - Fork 75
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Implement Texera User Avatars Featuer (#1373)
This PR creates an Angular component to show the avatar of a Texera user. The library/component **ngx-avatar** does not support retrieving Google users' avatars by Google ID (HaithemMosbahi/ngx-avatar#51), so I implemented the logic to retrieve Google users' avatars myself in the component. In order to send requests to Google People API, a public key is needed to provide. Creating a Google API Public Key is easy, please follow this instruction. https://developers.google.com/maps/documentation/maps-static/get-api-key Then fill the public key in the **environment.default.ts** `google: { clientID: "", publicKey: "", },` Demo: If the user is a normal Texera user, the avatar will be the default avatar with a random background color. 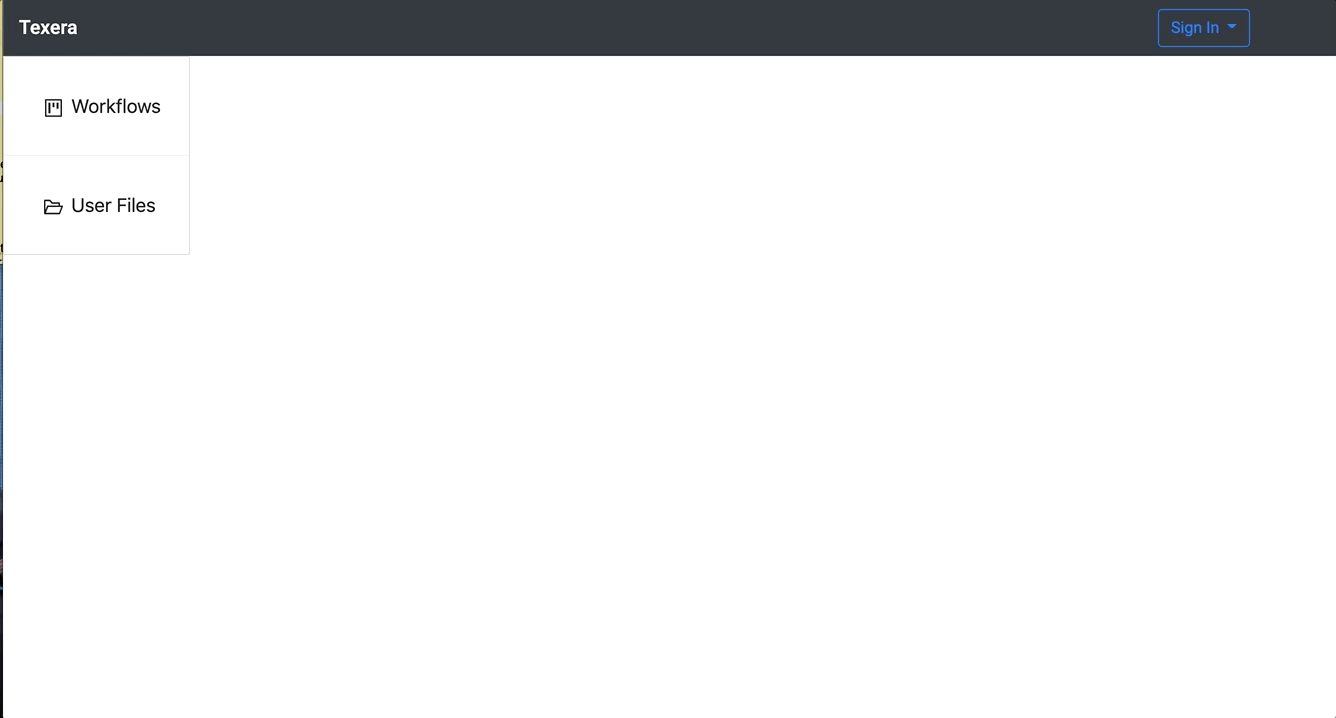 If the user is a Google user, the avatar will be its Google profile picture. 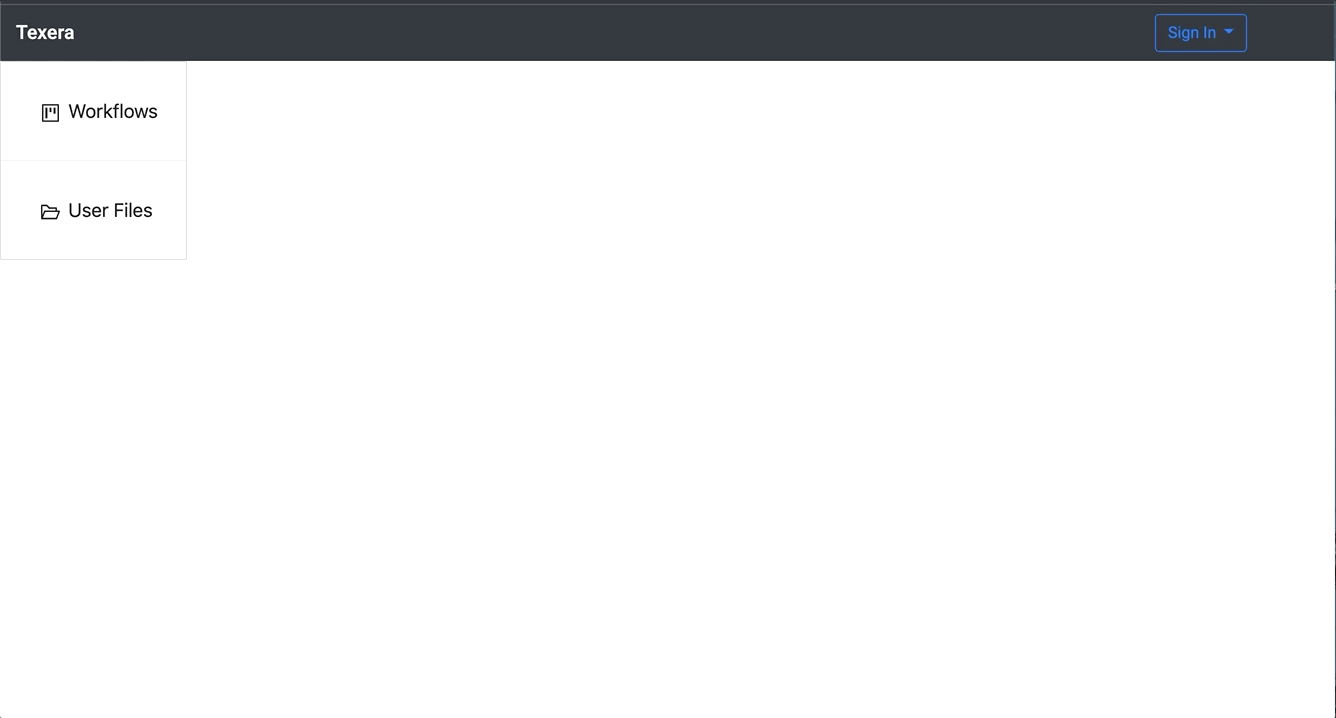 Co-authored-by: Yicong Huang <[email protected]>
- Loading branch information
1 parent
0edbbda
commit 363f683
Showing
13 changed files
with
184 additions
and
2 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
|
@@ -7,4 +7,5 @@ export interface User | |
extends Readonly<{ | ||
name: string; | ||
uid: number; | ||
googleId?: string; | ||
}> {} |
13 changes: 12 additions & 1 deletion
13
core/new-gui/src/app/dashboard/component/top-bar/user-icon/user-icon.component.html
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
10 changes: 10 additions & 0 deletions
10
core/new-gui/src/app/dashboard/component/user-avatar/user-avatar.component.html
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,10 @@ | ||
<div class="d-inline-block"> | ||
<nz-avatar | ||
id="texera-user-avatar" | ||
class="texera-user-avatar" | ||
[nzSrc]="googleUserAvatarSrc || ''" | ||
[nzAlt]="" | ||
[nzText]="abbreviate(userName || '')" | ||
> | ||
</nz-avatar> | ||
</div> |
16 changes: 16 additions & 0 deletions
16
core/new-gui/src/app/dashboard/component/user-avatar/user-avatar.component.scss
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,16 @@ | ||
.texera-user-avatar { | ||
object-fit: cover; | ||
border-radius: 50%; | ||
width: 38px; | ||
height: 38px; | ||
vertical-align: top; | ||
font-size: smaller; | ||
font-family: roboto, arial; | ||
color: white; | ||
line-height: 38px; | ||
text-align: center; | ||
} | ||
|
||
.texera-user-avatar:hover { | ||
box-shadow: #ccc 0px 0px 10px; | ||
} |
28 changes: 28 additions & 0 deletions
28
core/new-gui/src/app/dashboard/component/user-avatar/user-avatar.component.spec.ts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,28 @@ | ||
import { ComponentFixture, TestBed } from "@angular/core/testing"; | ||
import { HttpClientModule } from "@angular/common/http"; | ||
import { UserAvatarComponent } from "./user-avatar.component"; | ||
import { HttpClientTestingModule } from "@angular/common/http/testing"; | ||
|
||
describe("UserIconComponent", () => { | ||
let component: UserAvatarComponent; | ||
let fixture: ComponentFixture<UserAvatarComponent>; | ||
|
||
beforeEach(async () => { | ||
await TestBed.configureTestingModule({ | ||
declarations: [UserAvatarComponent], | ||
imports: [HttpClientModule, HttpClientTestingModule], | ||
}).compileComponents(); | ||
}); | ||
|
||
beforeEach(() => { | ||
fixture = TestBed.createComponent(UserAvatarComponent); | ||
component = fixture.componentInstance; | ||
component.googleId = undefined; | ||
component.userName = "fake Texera user"; | ||
fixture.detectChanges(); | ||
}); | ||
|
||
it("should create", () => { | ||
expect(component).toBeTruthy(); | ||
}); | ||
}); |
72 changes: 72 additions & 0 deletions
72
core/new-gui/src/app/dashboard/component/user-avatar/user-avatar.component.ts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,72 @@ | ||
import { GooglePeopleApiResponse } from "../../type/google-api-response"; | ||
import { Component, OnInit, Input } from "@angular/core"; | ||
import { UntilDestroy, untilDestroyed } from "@ngneat/until-destroy"; | ||
import { HttpClient } from "@angular/common/http"; | ||
import { environment } from "../../../../environments/environment"; | ||
|
||
@UntilDestroy() | ||
@Component({ | ||
selector: "texera-user-avatar", | ||
templateUrl: "./user-avatar.component.html", | ||
styleUrls: ["./user-avatar.component.scss"], | ||
}) | ||
|
||
/** | ||
* UserAvatarComponent is used to show the avatar of a user | ||
* The avatar of a Google user will be its Google profile picture | ||
* The avatar of a normal user will be a default one with the initial | ||
* | ||
* Use Google People API to retrieve google user's profile picture | ||
* Check https://developers.google.com/people/api/rest/v1/people/get for more details of the api usage | ||
* | ||
* @author Zhen Guan | ||
*/ | ||
export class UserAvatarComponent implements OnInit { | ||
public googleUserAvatarSrc: string = ""; | ||
private publicKey = environment.google.publicKey; | ||
|
||
constructor(private http: HttpClient) {} | ||
|
||
@Input() googleId?: string; | ||
@Input() userName?: string; | ||
|
||
ngOnInit(): void { | ||
if (!this.googleId && !this.userName) { | ||
throw new Error("google Id or user name should be provided"); | ||
} else if (this.googleId) { | ||
this.userName = ""; | ||
// get the avatar of the google user | ||
const googlePeopleAPIUrl = `https://people.googleapis.com/v1/people/${this.googleId}?personFields=names%2Cphotos&key=${this.publicKey}`; | ||
this.http | ||
.get<GooglePeopleApiResponse>(googlePeopleAPIUrl) | ||
.pipe(untilDestroyed(this)) | ||
.subscribe(res => { | ||
this.googleUserAvatarSrc = res.photos[0].url; | ||
}); | ||
} else { | ||
const r = Math.floor(Math.random() * 255); | ||
const g = Math.floor(Math.random() * 255); | ||
const b = Math.floor(Math.random() * 255); | ||
const avatar = document.getElementById("texera-user-avatar"); | ||
if (avatar) { | ||
avatar.style.backgroundColor = "rgba(" + r + "," + g + "," + b + ",0.8)"; | ||
} | ||
} | ||
} | ||
|
||
/** | ||
* abbreviates the name under 5 chars | ||
* @param userName | ||
*/ | ||
public abbreviate(userName: string): string { | ||
if (userName.length <= 5) { | ||
return userName; | ||
} else { | ||
return this.getUserInitial(userName).slice(0, 5); | ||
} | ||
} | ||
|
||
public getUserInitial(userName: string): string { | ||
return userName + "he"; | ||
} | ||
} |
24 changes: 24 additions & 0 deletions
24
core/new-gui/src/app/dashboard/type/google-api-response.ts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,24 @@ | ||
export interface Source | ||
extends Readonly<{ | ||
type: string; | ||
id: string; | ||
}> {} | ||
|
||
export interface Metadata | ||
extends Readonly<{ | ||
primary: boolean; | ||
source: Source; | ||
}> {} | ||
|
||
export interface Photo | ||
extends Readonly<{ | ||
metadata: Metadata; | ||
url: string; | ||
}> {} | ||
|
||
export interface GooglePeopleApiResponse | ||
extends Readonly<{ | ||
resourceName: string; | ||
etag: string; | ||
photos: Photo[]; | ||
}> {} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
|
@@ -77,6 +77,7 @@ export const defaultEnvironment = { | |
*/ | ||
google: { | ||
clientID: "", | ||
publicKey: "", | ||
}, | ||
|
||
/** | ||
|