You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
If my index.js file looks like this, my app behaves correctly in all 3 browsers:
// Works as expected in all 3 browsers
function MessageBlock()
{
return {
message: 'Hello World!',
changeMessage()
{
this.message = 'You changed the message from a function inside the data object!';
}
}
}
var data = MessageBlock();
rivets.bind(document.querySelector('#messages-block'),
{
data: data,
});
The result looks like this:
But, if I switch from using a factory function to a class, it stops working in Firefox and Edge.
index.js:
// Only works in Chrome
class MessageBlock
{
message = 'Hello World!';
changeMessage()
{
this.message = 'You changed the message from a class!';
}
}
var data = new MessageBlock();
rivets.bind(document.querySelector('#messages-block'),
{
data: data,
});
Why would I even want to use a class over a factory function, anyway?
I plan to eventually move to Typescript, and I want all of the data object's members to be type-checked. I can do that pretty easily with a class:
class MessageBlock
{
message: string = 'Hello World!';
changeMessage(): void
{
this.message = 'You changed the message from a class!';
}
}
But if I want to do the same thing with a factory function, I'd have to violate the DRY principle:
interface IMessageBlock
{
message: string;
changeMessage: () => void;
}
function MessageBlock(): IMessageBlock
{
return {
message: 'Hello World!',
changeMessage()
{
this.message = 'You changed the message from a function inside the data object!';
}
}
}
The text was updated successfully, but these errors were encountered:
The scenario
I have an index.html page that looks like this:
If my index.js file looks like this, my app behaves correctly in all 3 browsers:
The result looks like this:
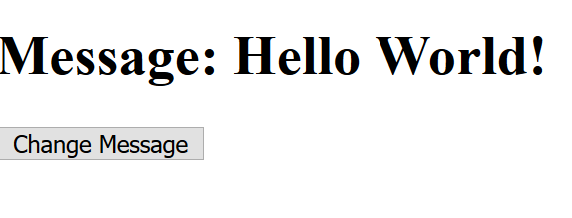
But, if I switch from using a factory function to a class, it stops working in Firefox and Edge.
index.js:
Why would I even want to use a class over a factory function, anyway?
I plan to eventually move to Typescript, and I want all of the data object's members to be type-checked. I can do that pretty easily with a class:
But if I want to do the same thing with a factory function, I'd have to violate the DRY principle:
The text was updated successfully, but these errors were encountered: